Thanks for visiting my blog!
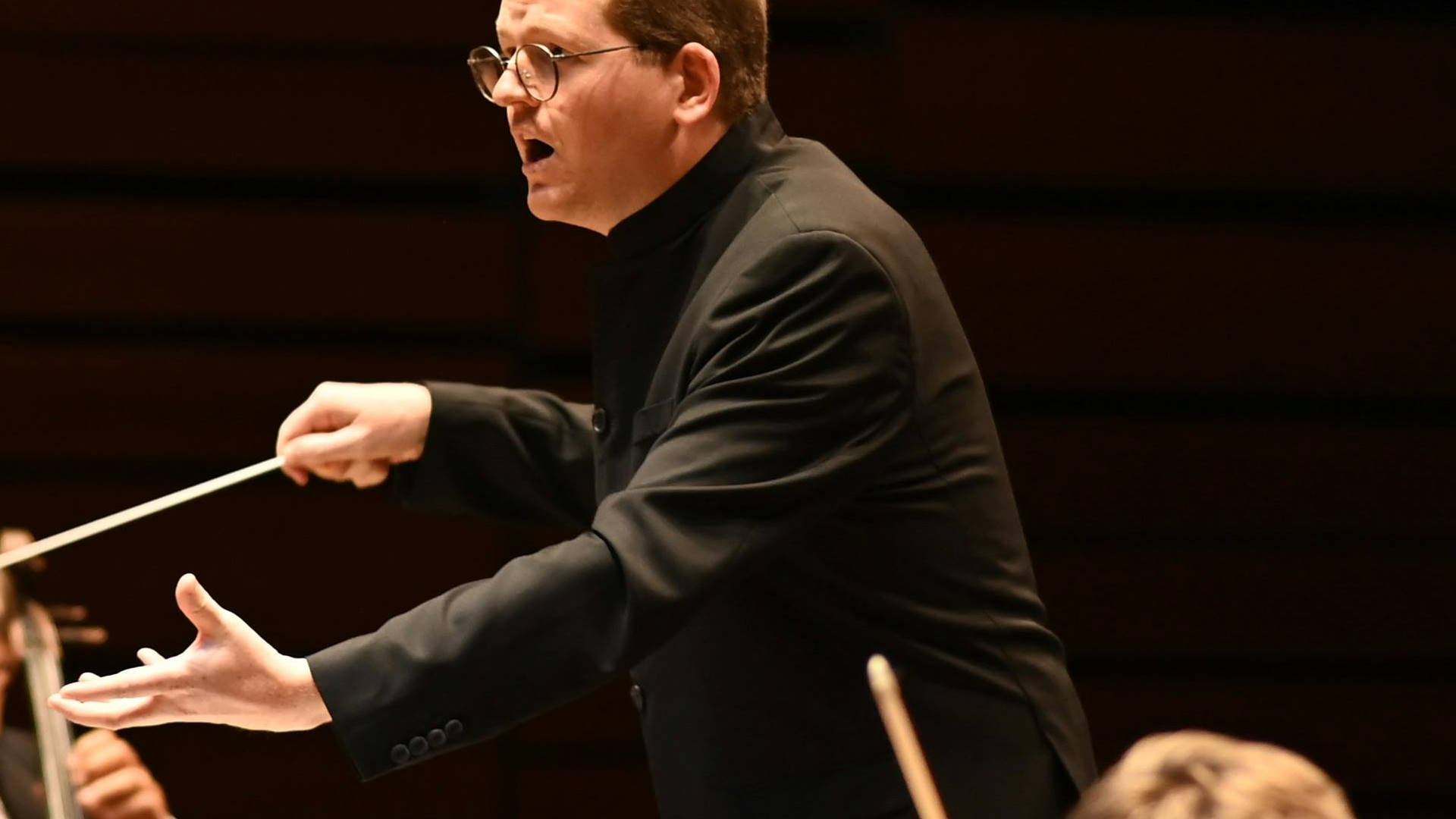
At one of my clients (he’ll be thrilled he made it in another blog post), I was showing him how to structure a complex Linq query. This came as a surprise to him and I thought it was worth a quick blog entry.
We’ve all been taught how Linq queries should look (using the Query syntax):
// Query Syntax
IEnumerable<int> numQuery1 =
from num in numbers
where num % 2 == 0
orderby num
select num;
This works fine, but like many of us, we’re used to the method syntax:
// Method Syntax
IEnumerable<int> numQuery1 = numbers
.Where(n => n % 2 == 0)
.OrderBy(n => n)
.ToList();
They both accomplish the same thing but I tend to prefer the method syntax. For me, the biggest difference is being able to compose the query. What I mean is this:
// Composing Linq
var qry = numbers.Where(n => n % 2 == 0);
if (excludeFours)
{
// Extend the Query
qry = qry.Where(n => n % 4 != 0);
}
// Add more linq operations
qry = qry.OrderBy(n => n);
var noFours = qry.ToList();
I think this is useful in a couple of ways. First, when you need to modify a query from input, this is less clunky that two completely different queries. But, more importantly I think, by breaking up a complex query into individual steps, it can help the readability of the query. For example:
// Using Entity Framework
IQueryable<Order> query = ctx.Orders
.Include(o => o.Items);
if (dateOrder) query = query.OrderByDescending(o => o.OrderDate);
var result = await query.ToListAsync();
While I think we’ve failed at talking about how linq is really working, I’m hoping this helps a little bit.
